C Data Types
C operators.
- C Input and Output
- C Control Flow

C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
Assignment operators in c.
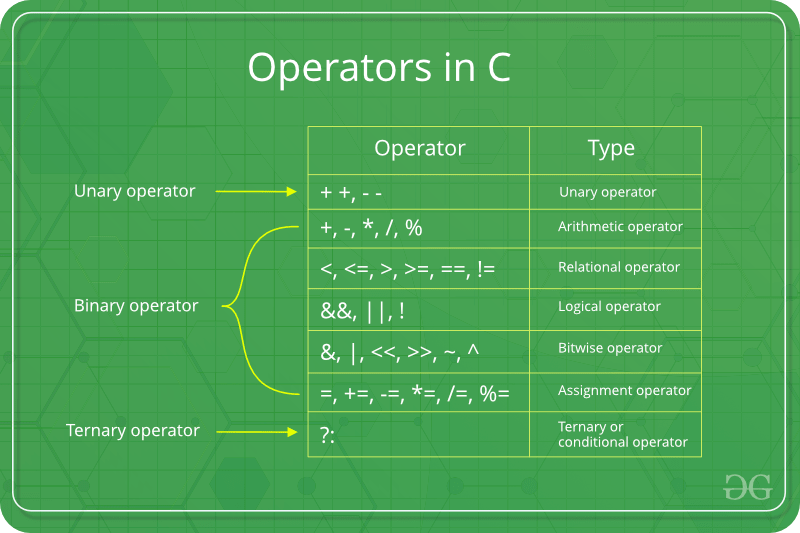
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Similar Reads
- C Programming Language Tutorial In this C Tutorial, you’ll learn all C programming basic to advanced concepts like variables, arrays, pointers, strings, loops, etc. This C Programming Tutorial is designed for both beginners as well as experienced professionals, who’re looking to learn and enhance their knowledge of the C programmi 8 min read
- C Language Introduction C is a procedural programming language initially developed by Dennis Ritchie in the year 1972 at Bell Laboratories of AT&T Labs. It was mainly developed as a system programming language to write the UNIX operating system. The main features of the C language include: General Purpose and PortableL 6 min read
- Features of C Programming Language C is a procedural programming language. It was initially developed by Dennis Ritchie in the year 1972. It was mainly developed as a system programming language to write an operating system. The main features of C language include low-level access to memory, a simple set of keywords, and a clean styl 3 min read
- C Programming Language Standard Introduction:The C programming language has several standard versions, with the most commonly used ones being C89/C90, C99, C11, and C18. C89/C90 (ANSI C or ISO C) was the first standardized version of the language, released in 1989 and 1990, respectively. This standard introduced many of the featur 6 min read
- C Hello World Program The “Hello World” program is the first step towards learning any programming language and also one of the simplest programs you will learn. To print the "Hello World", we can use the printf function from the stdio.h library that prints the given string on the screen. C Program to Print "Hello World" 2 min read
- Compiling a C Program: Behind the Scenes The compilation is the process of converting the source code of the C language into machine code. As C is a mid-level language, it needs a compiler to convert it into an executable code so that the program can be run on our machine. The C program goes through the following phases during compilation: 4 min read
- C Comments The comments in C are human-readable explanations or notes in the source code of a C program. A comment makes the program easier to read and understand. These are the statements that are not executed by the compiler or an interpreter. It is considered to be a good practice to document our code using 3 min read
- Tokens in C A token in C can be defined as the smallest individual element of the C programming language that is meaningful to the compiler. It is the basic component of a C program. Types of Tokens in CThe tokens of C language can be classified into six types based on the functions they are used to perform. Th 5 min read
- Keywords in C In C Programming language, there are many rules so to avoid different types of errors. One of such rule is not able to declare variable names with auto, long, etc. This is all because these are keywords. Let us check all keywords in C language. What are Keywords?Keywords are predefined or reserved w 13 min read
C Variables and Constants
- C Variables A variable in C language is the name associated with some memory location to store data of different types. There are many types of variables in C depending on the scope, storage class, lifetime, type of data they store, etc. A variable is the basic building block of a C program that can be used in 9 min read
- Constants in C The constants in C are the read-only variables whose values cannot be modified once they are declared in the C program. The type of constant can be an integer constant, a floating pointer constant, a string constant, or a character constant. In C language, the const keyword is used to define the con 6 min read
- Const Qualifier in C The qualifier const can be applied to the declaration of any variable to specify that its value will not be changed (which depends upon where const variables are stored, we may change the value of the const variable by using a pointer). The result is implementation-defined if an attempt is made to c 7 min read
- Different ways to declare variable as constant in C There are many different ways to make the variable as constant in C. Some of the popular ones are: Using const KeywordUsing MacrosUsing enum Keyword1. Using const KeywordThe const keyword specifies that a variable or object value is constant and can't be modified at the compilation time. Syntaxconst 2 min read
- Scope rules in C The scope of a variable in C is the block or the region in the program where a variable is declared, defined, and used. Outside this region, we cannot access the variable, and it is treated as an undeclared identifier. The scope is the area under which a variable is visible.The scope of an identifie 6 min read
- Internal Linkage and External Linkage in C It is often quite hard to distinguish between scope and linkage, and the roles they play. This article focuses on scope and linkage, and how they are used in C language. Note: All C programs have been compiled on 64 bit GCC 4.9.2. Also, the terms "identifier" and "name" have been used interchangeabl 9 min read
- Global Variables in C Prerequisite: Variables in C In a programming language, each variable has a particular scope attached to them. The scope is either local or global. This article will go through global variables, their advantages, and their properties. The Declaration of a global variable is very similar to that of a 3 min read
- Data Types in C Each variable in C has an associated data type. It specifies the type of data that the variable can store like integer, character, floating, double, etc. Each data type requires different amounts of memory and has some specific operations which can be performed over it. The data types in C can be cl 7 min read
- Literals in C In C, Literals are the constant values that are assigned to the variables. Literals represent fixed values that cannot be modified. Literals contain memory but they do not have references as variables. Generally, both terms, constants, and literals are used interchangeably. For example, “const int = 4 min read
- Escape Sequence in C The escape sequence in C is the characters or the sequence of characters that can be used inside the string literal. The purpose of the escape sequence is to represent the characters that cannot be used normally using the keyboard. Some escape sequence characters are the part of ASCII charset but so 6 min read
- bool in C The bool in C is a fundamental data type in most that can hold one of two values: true or false. It is used to represent logical values and is commonly used in programming to control the flow of execution in decision-making statements such as if-else statements, while loops, and for loops. In this a 6 min read
- Integer Promotions in C Some data types like char , short int take less number of bytes than int, these data types are automatically promoted to int or unsigned int when an operation is performed on them. This is called integer promotion. For example no arithmetic calculation happens on smaller types like char, short and e 2 min read
- Character Arithmetic in C As already known character range is between -128 to 127 or 0 to 255. This point has to be kept in mind while doing character arithmetic. What is Character Arithmetic?Character arithmetic is used to implement arithmetic operations like addition, subtraction, multiplication, and division on characters 2 min read
- Type Conversion in C Type conversion in C is the process of converting one data type to another. The type conversion is only performed to those data types where conversion is possible. Type conversion is performed by a compiler. In type conversion, the destination data type can't be smaller than the source data type. Ty 5 min read
C Input/Output
- Basic Input and Output in C C language has standard libraries that allow input and output in a program. The stdio.h or standard input output library in C that has methods for input and output. scanf()The scanf() method, in C, reads the value from the console as per the type specified and store it in the given address. Syntax: 3 min read
- Format Specifiers in C The format specifier in C is used to tell the compiler about the type of data to be printed or scanned in input and output operations. They always start with a % symbol and are used in the formatted string in functions like printf(), scanf, sprintf(), etc. The C language provides a number of format 6 min read
- printf in C In C language, printf() function is used to print formatted output to the standard output stdout (which is generally the console screen). The printf function is a part of the C standard library <stdio.h> and it can allow formatting the output in numerous ways. Syntax of printfprintf ( "formatt 5 min read
- scanf in C In C programming language, scanf is a function that stands for Scan Formatted String. It is used to read data from stdin (standard input stream i.e. usually keyboard) and then writes the result into the given arguments. It accepts character, string, and numeric data from the user using standard inpu 2 min read
- Scansets in C scanf family functions support scanset specifiers which are represented by %[]. Inside scanset, we can specify single character or range of characters. While processing scanset, scanf will process only those characters which are part of scanset. We can define scanset by putting characters inside squ 2 min read
- Formatted and Unformatted Input/Output functions in C with Examples This article focuses on discussing the following topics in detail- Formatted I/O Functions.Unformatted I/O Functions.Formatted I/O Functions vs Unformatted I/O Functions.Formatted I/O Functions Formatted I/O functions are used to take various inputs from the user and display multiple outputs to the 9 min read
- Operators in C In C language, operators are symbols that represent operations to be performed on one or more operands. They are the basic components of the C programming. In this article, we will learn about all the built-in operators in C with examples. What is a C Operator?An operator in C can be defined as the 14 min read
- Arithmetic Operators in C Arithmetic Operators are the type of operators in C that are used to perform mathematical operations in a C program. They can be used in programs to define expressions and mathematical formulas. What are C Arithmetic Operators?The C arithmetic operators are the symbols that are used to perform mathe 7 min read
- Unary operators in C Unary operators are the operators that perform operations on a single operand to produce a new value. Types of unary operatorsTypes of unary operators are mentioned below: Unary minus ( - )Increment ( ++ )Decrement ( -- )NOT ( ! )Addressof operator ( & )sizeof()1. Unary MinusThe minus operator ( 5 min read
- Relational Operators in C In C, relational operators are the symbols that are used for comparison between two values to understand the type of relationship a pair of numbers shares. The result that we get after the relational operation is a boolean value, that tells whether the comparison is true or false. Relational operato 4 min read
- Bitwise Operators in C In C, the following 6 operators are bitwise operators (also known as bit operators as they work at the bit-level). They are used to perform bitwise operations in C. The & (bitwise AND) in C takes two numbers as operands and does AND on every bit of two numbers. The result of AND is 1 only if bot 7 min read
- C Logical Operators Logical operators in C are used to combine multiple conditions/constraints. Logical Operators returns either 0 or 1, it depends on whether the expression result is true or false. In C programming for decision-making, we use logical operators. We have 3 logical operators in the C language: Logical AN 6 min read
- Assignment Operators in C Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compi 3 min read
- Increment and Decrement Operators in C The increment ( ++ ) and decrement ( -- ) operators in C are unary operators for incrementing and decrementing the numeric values by 1 respectively. The incrementation and decrementation are one of the most frequently used operations in programming for looping, array traversal, pointer arithmetic, a 4 min read
- Conditional or Ternary Operator (?:) in C The conditional operator in C is kind of similar to the if-else statement as it follows the same algorithm as of if-else statement but the conditional operator takes less space and helps to write the if-else statements in the shortest way possible. It is also known as the ternary operator in C as it 3 min read
- sizeof operator in C Sizeof is a much-used operator in the C. It is a compile-time unary operator which can be used to compute the size of its operand. The result of sizeof is of the unsigned integral type which is usually denoted by size_t. sizeof can be applied to any data type, including primitive types such as integ 4 min read
- Operator Precedence and Associativity in C The concept of operator precedence and associativity in C helps in determining which operators will be given priority when there are multiple operators in the expression. It is very common to have multiple operators in C language and the compiler first evaluates the operater with higher precedence. 8 min read
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if ) The conditional statements (also known as decision control structures) such as if, if else, switch, etc. are used for decision-making purposes in C programs. They are also known as Decision-Making Statements and are used to evaluate one or more conditions and make the decision whether to execute a s 11 min read
- C - if Statement The if in C is the most simple decision-making statement. It consists of the test condition and if block or body. If the given condition is true only then the if block will be executed. What is if in C? The if in C is a decision-making statement that is used to execute a block of code based on the v 5 min read
- C if...else Statement The if-else statement in C is a flow control statement used for decision-making in the C program. It is one of the core concepts of C programming. It is an extension of the if in C that includes an else block along with the already existing if block. C if Statement The if statement in C is used to e 6 min read
- C if else if ladder if else if ladder in C programming is used to test a series of conditions sequentially. Furthermore, if a condition is tested only when all previous if conditions in the if-else ladder are false. If any of the conditional expressions evaluate to be true, the appropriate code block will be executed, 3 min read
- Switch Statement in C Switch case statement evaluates a given expression and based on the evaluated value(matching a certain condition), it executes the statements associated with it. Basically, it is used to perform different actions based on different conditions(cases). Switch case statements follow a selection-control 8 min read
- Using Range in switch Case in C You all are familiar with switch case in C, but did you know you can use a range of numbers instead of a single number or character in the case statement? Range in switch case can be useful when we want to run the same set of statements for a range of numbers so that we do not have to write cases se 2 min read
- C - Loops Loops in programming are used to repeat a block of code until the specified condition is met. A loop statement allows programmers to execute a statement or group of statements multiple times without repetition of code. [GFGTABS] C // C program to illustrate need of loops #include <stdio.h> int 6 min read
- C for Loop In C programming, loops are responsible for performing repetitive tasks using a short code block that executes until the condition holds true. In this article, we will learn about for loop in C. for Loop in CThe for loop in C Language provides a functionality/feature to repeat a set of statements a 6 min read
- while loop in C The while Loop is an entry-controlled loop in C programming language. This loop can be used to iterate a part of code while the given condition remains true. Syntax The while loop syntax is as follows: while (test expression) { // body consisting of multiple statements }Example The below example sho 3 min read
- do...while Loop in C Loops in C language are the control flow statements that are used to repeat some part of the code till the given condition is satisfied. The do-while loop is one of the three loop statements in C, the others being while loop and for loop. It is mainly used to traverse arrays, vectors, and other data 7 min read
- For Versus While Question: Is there any example for which the following two loops will not work the same way? [GFGTABS] C /*Program 1 --> For loop*/ for (<init - stmnt>;<boolean - expr>;<incr - stmnt>) { <body-statements> } /*Program 2 --> While loop*/ <init - stmnt>; while (<b 2 min read
- Continue Statement in C The continue statement in C is a jump statement that is used to bring the program control to the start of the loop. We can use the continue statement in the while loop, for loop, or do..while loop to alter the normal flow of the program execution. Unlike break, it cannot be used with a C switch case 5 min read
- Break Statement in C The break statement is one of the four jump statements in the C language. The purpose of the break statement in C is for unconditional exit from the loop What is break in C?The break in C is a loop control statement that breaks out of the loop when encountered. It can be used inside loops or switch 6 min read
- goto Statement in C The C goto statement is a jump statement which is sometimes also referred to as an unconditional jump statement. The goto statement can be used to jump from anywhere to anywhere within a function. Syntax: Syntax1 | Syntax2----------------------------goto label; | label: . | .. | .. | .label: | goto 3 min read
- C Functions A function in C is a set of statements that when called perform some specific tasks. It is the basic building block of a C program that provides modularity and code reusability. The programming statements of a function are enclosed within { } braces, having certain meanings and performing certain op 10 min read
- User-Defined Function in C A user-defined function is a type of function in C language that is defined by the user himself to perform some specific task. It provides code reusability and modularity to our program. User-defined functions are different from built-in functions as their working is specified by the user and no hea 6 min read
- Parameter Passing Techniques in C In C, there are different ways in which parameter data can be passed into and out of methods and functions. Let us assume that a function B() is called from another function A(). In this case, A is called the "caller function" and B is called the "called function or callee function". Also, the argum 5 min read
- Function Prototype in C The C function prototype is a statement that tells the compiler about the function's name, its return type, numbers and data types of its parameters. By using this information, the compiler cross-checks function parameters and their data type with function definition and function call. Function prot 5 min read
- How can I return multiple values from a function? We all know that a function in C can return only one value. So how do we achieve the purpose of returning multiple values. Well, first take a look at the declaration of a function. int foo(int arg1, int arg2); So we can notice here that our interface to the function is through arguments and return v 2 min read
- main Function in C The main function is an integral part of the programming languages such as C, C++, and Java. The main function in C is the entry point of a program where the execution of a program starts. It is a user-defined function that is mandatory for the execution of a program because when a C program is exec 4 min read
- Implicit return type int in C Predict the output of following C program. #include <stdio.h> fun(int x) { return x*x; } int main(void) { printf("%d", fun(10)); return 0; } Output: 100 The important thing to note is, there is no return type for fun(), the program still compiles and runs fine in most of the C compil 1 min read
- Callbacks in C A callback is any executable code that is passed as an argument to another code, which is expected to call back (execute) the argument at a given time. In simple language, If a reference of a function is passed to another function as an argument to call it, then it will be called a Callback function 2 min read
- Nested functions in C Some programmer thinks that defining a function inside an another function is known as "nested function". But the reality is that it is not a nested function, it is treated as lexical scoping. Lexical scoping is not valid in C because the compiler cant reach/find the correct memory location of the i 2 min read
- Variadic functions in C Variadic functions are functions that can take a variable number of arguments. In C programming, a variadic function adds flexibility to the program. It takes one fixed argument and then any number of arguments can be passed. The variadic function consists of at least one fixed variable and then an 3 min read
- _Noreturn function specifier in C The _Noreturn keyword appears in a function declaration and specifies that the function does not return by executing the return statement or by reaching the end of the function body. If the function declared _Noreturn returns, the behavior is undefined. A compiler diagnostic is recommended if this c 2 min read
- Predefined Identifier __func__ in C Before we start discussing __func__, let us write some code snippets and anticipate the output: C/C++ Code // C program to demonstrate working of a // Predefined Identifier __func__ #include <stdio.h> int main() { // %s indicates that the program will read strings printf("%s", __func 2 min read
- C Library math.h Functions The math.h header defines various C mathematical functions and one macro. All the functions available in this library take double as an argument and return double as the result. Let us discuss some important C math functions one by one. C Math Functions1. double ceil (double x) The C library functio 6 min read
C Arrays & Strings
- C Arrays Array in C is one of the most used data structures in C programming. It is a simple and fast way of storing multiple values under a single name. In this article, we will study the different aspects of array in C language such as array declaration, definition, initialization, types of arrays, array s 15+ min read
- Properties of Array in C An array in C is a fixed-size homogeneous collection of elements stored at a contiguous memory location. It is a derived data type in C that can store elements of different data types such as int, char, struct, etc. It is one of the most popular data types widely used by programmers to solve differe 8 min read
- Multidimensional Arrays in C - 2D and 3D Arrays Prerequisite: Arrays in C A multi-dimensional array can be defined as an array that has more than one dimension. Having more than one dimension means that it can grow in multiple directions. Some popular multidimensional arrays are 2D arrays and 3D arrays. In this article, we will learn about multid 10 min read
- Initialization of Multidimensional Array in C In C, initialization of a multidimensional array can have left most dimensions as optional. Except for the leftmost dimension, all other dimensions must be specified. For example, the following program fails in compilation because two dimensions are not specified. C/C++ Code #include <stdio.h> 2 min read
- Pass Array to Functions in C In C, the whole array cannot be passed as an argument to a function. However, you can pass a pointer to an array without an index by specifying the array's name. Arrays in C are always passed to the function as pointers pointing to the first element of the array. SyntaxIn C, we have three ways to pa 6 min read
- How to pass a 2D array as a parameter in C? This post is an extension of How to dynamically allocate a 2D array in C? A one dimensional array can be easily passed as a pointer, but syntax for passing a 2D array to a function can be difficult to remember. One important thing for passing multidimensional arrays is, first array dimension does no 4 min read
- What are the data types for which it is not possible to create an array? In C, it is possible to have array of all types except following. 1) void. 2) functions. For example, below program throws compiler error int main() { void arr[100]; } Output: error: declaration of 'arr' as array of voids But we can have array of void pointers and function pointers. The below progra 1 min read
- How to pass an array by value in C ? In C, array name represents address and when we pass an array, we actually pass address and the parameter receiving function always accepts them as pointers (even if we use [], refer this for details). How to pass array by value, i.e., how to make sure that we have a new copy of array when we pass i 2 min read
- Strings in C A String in C programming is a sequence of characters terminated with a null character '\0'. The C String is stored as an array of characters. The difference between a character array and a C string is that the string in C is terminated with a unique character '\0'. C String Declaration SyntaxDeclar 8 min read
- Array of Strings in C In C programming String is a 1-D array of characters and is defined as an array of characters. But an array of strings in C is a two-dimensional array of character types. Each String is terminated with a null character (\0). It is an application of a 2d array. Syntax: char variable_name[r][m] = {lis 3 min read
- What is the difference between single quoted and double quoted declaration of char array? In C, when a character array is initialized with a double-quoted string and the array size is not specified, the compiler automatically allocates one extra space for string terminator '\0'. Example The below example demonstrates the initialization of a char array with a double-quoted string without 2 min read
- C String Functions The C string functions are built-in functions that can be used for various operations and manipulations on strings. These string functions can be used to perform tasks such as string copy, concatenation, comparison, length, etc. The <string.h> header file contains these string functions. In th 8 min read
- C Pointers A pointer is defined as a derived data type that can store the memory address of other variables, functions, or even other pointers. It is one of the core components of the C programming language allowing low-level memory access, dynamic memory allocation, and many other functionalities in C. Syntax 10 min read
- Pointer Arithmetics in C with Examples Pointer Arithmetic is the set of valid arithmetic operations that can be performed on pointers. The pointer variables store the memory address of another variable. It doesn't store any value. Hence, there are only a few operations that are allowed to perform on Pointers in C language. The C pointer 10 min read
- C - Pointer to Pointer (Double Pointer) Prerequisite: Pointers in C The pointer to a pointer in C is used when we want to store the address of another pointer. The first pointer is used to store the address of the variable. And the second pointer is used to store the address of the first pointer. That is why they are also known as double- 5 min read
- Function Pointer in C In C, like normal data pointers (int *, char *, etc), we can have pointers to functions. Following is a simple example that shows declaration and function call using function pointer. Let's take a look at an example: [GFGTABS] C #include <stdio.h> void fun(int a) { printf("Value of a is % 5 min read
- How to declare a pointer to a function? While a pointer to a variable or an object is used to access them indirectly, a pointer to a function is used to invoke a function indirectly. Well, we assume that you know what it means by a pointer in C. So how do we create a pointer to an integer in C? Huh..it is pretty simple... int *ptrInteger; 2 min read
- Pointer to an Array | Array Pointer A pointer to an array is a pointer that points to the whole array instead of the first element of the array. It considers the whole array as a single unit instead of it being a collection of given elements. Consider the following example: [GFGTABS] C #include<stdio.h> int main() { int arr[5] = 5 min read
- Difference between constant pointer, pointers to constant, and constant pointers to constants In this article, we will discuss the differences between constant pointer, pointers to constant & constant pointers to constants. Pointers are the variables that hold the address of some other variables, constants, or functions. There are several ways to qualify pointers using const. Pointers to 3 min read
- Pointer vs Array in C Most of the time, pointer and array accesses can be treated as acting the same, the major exceptions being: 1. the sizeof operator sizeof(array) returns the amount of memory used by all elements in the array sizeof(pointer) only returns the amount of memory used by the pointer variable itself 2. the 1 min read
- Dangling, Void , Null and Wild Pointers in C In C programming pointers are used to manipulate memory addresses, to store the address of some variable or memory location. But certain situations and characteristics related to pointers become challenging in terms of memory safety and program behavior these include Dangling (when pointing to deall 6 min read
- Near, Far and Huge Pointers in C In older times, the intel processors had 16-bit registers but the address bus was 20-bits wide. Due to this, the registers were not able to hold the entire address at once. As a solution, the memory was divided into segments of 64 kB size, and the near pointers, far pointers, and huge pointers were 4 min read
- restrict keyword in C In the C programming language (after the C99 standard), a new keyword is introduced known as restrict. restrict keyword is mainly used in pointer declarations as a type qualifier for pointers.It doesn't add any new functionality. It is only a way for the programmer to inform about an optimization th 2 min read
C User-Defined Data Types
- C Structures The structure in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its member and they can be of any valid data type. Ad 10 min read
- dot (.) Operator in C The C dot (.) operator is used for direct member selection via the name of variables of type struct and union. Also known as the direct member access operator, it is a binary operator that helps us to extract the value of members of the structures and unions. Syntax of Dot Operatorvariable_name.memb 2 min read
- C typedef The typedef is a keyword that is used to provide existing data types with a new name. The C typedef keyword is used to redefine the name of already existing data types. When names of datatypes become difficult to use in programs, typedef is used with user-defined datatypes, which behave similarly to 4 min read
- Structure Member Alignment, Padding and Data Packing In C, the structures are used as data packs. They don't provide any data encapsulation or data hiding features. In this article, we will discuss the property of structure padding in C along with data alignment and structure packing. Data Alignment in MemoryEvery data type in C will have alignment re 12 min read
- Flexible Array Members in a structure in C Flexible Array Member(FAM) is a feature introduced in the C99 standard of the C programming language. For the structures in C programming language from C99 standard onwards, we can declare an array without a dimension and whose size is flexible in nature.Such an array inside the structure should pre 4 min read
- C Unions The Union is a user-defined data type in C language that can contain elements of the different data types just like structure. But unlike structures, all the members in the C union are stored in the same memory location. Due to this, only one member can store data at the given instance. Syntax of Un 5 min read
- Bit Fields in C In C, we can specify the size (in bits) of the structure and union members. The idea of bit-field is to use memory efficiently when we know that the value of a field or group of fields will never exceed a limit or is within a small range. C Bit fields are used when the storage of our program is limi 8 min read
- Difference Between Structure and Union in C Structures in C is a user-defined data type available in C that allows to combining of data items of different kinds. Structures are used to represent a record. Defining a structure: To define a structure, you must use the struct statement. The struct statement defines a new data type, with more tha 4 min read
- Anonymous Union and Structure in C In C11 standard of C, anonymous Unions and structures were added. Anonymous unions/structures are also known as unnamed unions/structures as they don't have names. Since there is no names, direct objects(or variables) of them are not created and we use them in nested structure or unions. Definition 2 min read
- Enumeration (or enum) in C Enumeration (or enum) is a user defined data type in C. It is mainly used to assign names to integral constants, the names make a program easy to read and maintain. enum State {Working = 1, Failed = 0}; The keyword 'enum' is used to declare new enumeration types in C and C++. Enums in C allow you to 4 min read
C Storage Classes
- Storage Classes in C C Storage Classes are used to describe the features of a variable/function. These features basically include the scope, visibility, and lifetime which help us to trace the existence of a particular variable during the runtime of a program. C language uses 4 storage classes, namely: Storage classes i 7 min read
- extern Keyword in C extern keyword in C applies to C variables (data objects) and C functions. Basically, the extern keyword extends the visibility of the C variables and C functions. That's probably the reason why it was named extern. Though most people probably understand the difference between the "declaration" and 7 min read
- Static Variables in C Static variables have the property of preserving their value even after they are out of their scope! Hence, a static variable preserves its previous value in its previous scope and is not initialized again in the new scope. Syntax: static data_type var_name = var_value;Static variables in C retain t 4 min read
- Initialization of static variables in C In C, static variables can only be initialized using constant literals. For example, following program fails in compilation. [GFGTABS] C #include<stdio.h> int initializer(void) { return 50; } int main() { static int i = initializer(); printf(" value of i = %d", i); getchar(); return 1 min read
- Static functions in C In C, functions are global by default. The “static” keyword before a function name makes it static. For example, the below function fun() is static. [GFGTABS] C static int fun(void) { printf("I am a static function "); } [/GFGTABS]Unlike global functions in C, access to static functions is 2 min read
- Understanding "volatile" qualifier in C | Set 2 (Examples) The volatile keyword is intended to prevent the compiler from applying any optimizations on objects that can change in ways that cannot be determined by the compiler. Objects declared as volatile are omitted from optimization because their values can be changed by code outside the scope of current c 6 min read
- Understanding "register" keyword in C Registers are faster than memory to access, so the variables which are most frequently used in a C program can be put in registers using the register keyword. The keyword register hints to the compiler that a given variable can be put in a register. It's the compiler's choice to put it in a register 3 min read
C Memory Management
- Memory Layout of C Programs A typical memory representation of a C program consists of the following sections. Text segment (i.e. instructions)Initialized data segment Uninitialized data segment (bss)Heap Stack A typical memory layout of a running process 1. Text Segment: A text segment, also known as a code segment or simply 6 min read
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc() Since C is a structured language, it has some fixed rules for programming. One of them includes changing the size of an array. An array is a collection of items stored at contiguous memory locations. As can be seen, the length (size) of the array above is 9. But what if there is a requirement to cha 9 min read
- Difference Between malloc() and calloc() with Examples The functions malloc() and calloc() are library functions that allocate memory dynamically. Dynamic means the memory is allocated during runtime (execution of the program) from the heap segment. Initializationmalloc() allocates a memory block of given size (in bytes) and returns a pointer to the beg 3 min read
- What is Memory Leak? How can we avoid? A memory leak occurs when programmers create a memory in a heap and forget to delete it. The consequence of the memory leak is that it reduces the performance of the computer by reducing the amount of available memory. Eventually, in the worst case, too much of the available memory may become alloca 3 min read
- Dynamic Array in C Array in C is static in nature, so its size should be known at compile time and we can't change the size of the array after its declaration. Due to this, we may encounter situations where our array doesn't have enough space left for required elements or we allotted more than the required memory lead 9 min read
- How to dynamically allocate a 2D array in C? Following are different ways to create a 2D array on the heap (or dynamically allocate a 2D array).In the following examples, we have considered 'r' as number of rows, 'c' as number of columns and we created a 2D array with r = 3, c = 4 and the following values 1 2 3 4 5 6 7 8 9 10 11 12 1) Using a 5 min read
- Dynamically Growing Array in C Prerequisite: Dynamic Memory Allocation in C A Dynamically Growing Array is a type of dynamic array, which can automatically grow in size to store data. The C language only has static arrays whose size should be known at compile time and cannot be changed after declaration. This leads to problems li 7 min read
C Preprocessor
- C Preprocessors Preprocessors are programs that process the source code before compilation. Several steps are involved between writing a program and executing a program in C. Let us have a look at these steps before we actually start learning about Preprocessors. You can see the intermediate steps in the above diag 10 min read
- C Preprocessor Directives In almost every C program we come across, we see a few lines at the top of the program preceded by a hash (#) sign. They are called preprocessor directives and are preprocessed by the preprocessor before actual compilation begins. The end of these lines is identified by the newline character '\n', n 8 min read
- How a Preprocessor works in C? Compiling a C program - Behind the Scene A Preprocessor is a system software (a computer program that is designed to run on computer's hardware and application programs). It performs preprocessing of the High Level Language(HLL). Preprocessing is the first step of the language processing system. Lan 3 min read
- Header Files in C In C language, header files contain a set of predefined standard library functions. The .h is the extension of the header files in C and we request to use a header file in our program by including it with the C preprocessing directive "#include". C Header files offer the features like library functi 7 min read
- What’s difference between header files "stdio.h" and "stdlib.h" ? These are two important header files used in C programming. While “<stdio.h>” is header file for Standard Input Output, “<stdlib.h>” is header file for Standard Library. One easy way to differentiate these two header files is that “<stdio.h>” contains declaration of printf() and sc 2 min read
- How to write your own header file in C? As we all know that files with .h extension are called header files in C. These header files generally contain function declarations which we can be used in our main C program, like for e.g. there is need to include stdio.h in our C program to use function printf() in the program. So the question ar 4 min read
- Macros and its types in C In C, a macro is a piece of code in a program that is replaced by the value of the macro. Macro is defined by #define directive. Whenever a macro name is encountered by the compiler, it replaces the name with the definition of the macro. Macro definitions need not be terminated by a semi-colon(;). E 6 min read
- Interesting Facts about Macros and Preprocessors in C In a C program, all lines that start with # are processed by preprocessor which is a special program invoked by the compiler. by this we mean to say that the ‘#’ symbol is used to process the functionality prior than other statements in the program, that is, which means it processes some code before 6 min read
- # and ## Operators in C Stringizing operator (#)The stringizing operator (#) is a preprocessor operator that causes the corresponding actual argument to be enclosed in double quotation marks. The # operator, which is generally called the stringize operator, turns the argument it precedes into a quoted string. It is also kn 2 min read
- How to print a variable name in C? How to print and store a variable name in string variable? We strongly recommend you to minimize your browser and try this yourself first In C, there’s a # directive, also called ‘Stringizing Operator’, which does this magic. Basically # directive converts its argument in a string. #include <stdi 1 min read
- Multiline macros in C In this article, we will discuss how to write a multi-line macro. We can write multi-line macro same like function, but each statement ends with "\". Let us see with example. Below is simple macro, which accepts input number from user, and prints whether entered number is even or odd. #include <s 3 min read
- Variable length arguments for Macros Like functions, we can also pass variable length arguments to macros. For this we will use the following preprocessor identifiers.To support variable length arguments in macro, we must include ellipses (...) in macro definition. There is also "__VA_ARGS__" preprocessing identifier which takes care o 2 min read
- Branch prediction macros in GCC One of the most used optimization techniques in the Linux kernel is " __builtin_expect". When working with conditional code (if-else statements), we often know which branch is true and which is not. If compiler knows this information in advance, it can generate most optimized code. Let us see macro 2 min read
- typedef versus #define in C typedef: The typedef is used to give data type a new name. For example, // C program to demonstrate typedef #include <stdio.h> // After this line BYTE can be used // in place of unsigned char typedef unsigned char BYTE; int main() { BYTE b1, b2; b1 = 'c'; printf("%c ", b1); return 0; 3 min read
- Difference between #define and const in C? #define is a preprocessor directive. Data defined by the #define macro definition are preprocessed, so that your entire code can use it. This can free up space and increase compilation times.const variables are considered variables, and not macro definitions. Long story short: CONSTs are handled by 2 min read
- Basics of File Handling in C File handling in C is the process in which we create, open, read, write, and close operations on a file. C language provides different functions such as fopen(), fwrite(), fread(), fseek(), fprintf(), etc. to perform input, output, and many different C file operations in our program. Why do we need 15 min read
- C fopen() function with Examples The fopen() method in C is a library function that is used to open a file to perform various operations which include reading, writing, etc. along with various modes. If the file exists then the fopen() function opens the particular file else a new file is created. SyntaxThe syntax of C fopen() is: 5 min read
- EOF, getc() and feof() in C In C/C++, getc() returns the End of File (EOF) when the end of the file is reached. getc() also returns EOF when it fails. So, only comparing the value returned by getc() with EOF is not sufficient to check for the actual end of the file. To solve this problem, C provides feof() which returns a non- 3 min read
- fgets() and gets() in C language For reading a string value with spaces, we can use either gets() or fgets() in C programming language. Here, we will see what is the difference between gets() and fgets(). fgets()The fgets() reads a line from the specified stream and stores it into the string pointed to by str. It stops when either 3 min read
- fseek() vs rewind() in C fseek() and rewind() both functions are used to file positioning defined in <stdio.h> header file. In this article, we will discuss the differences in the usage and behavior of both functions. fseek() Function fseek() is used to set the file position indicator to a specified offset from the sp 3 min read
- What is return type of getchar(), fgetc() and getc() ? In C, return type of getchar(), fgetc() and getc() is int (not char). So it is recommended to assign the returned values of these functions to an integer type variable. char ch; /* May cause problems */ while ((ch = getchar()) != EOF) { putchar(ch); } Here is a version that uses integer to compare t 1 min read
- Read/Write Structure From/to a File in C For writing in the file, it is easy to write string or int to file using fprintf and putc, but you might have faced difficulty when writing contents of the struct. fwrite and fread make tasks easier when you want to write and read blocks of data. Writing Structure to a File using fwriteWe can use fw 3 min read
- C Program to print contents of file In C, reading the contents of a file involves opening the file, reading its data, and then processing or displaying the data. Example Input: File containing “This is a test file.\nIt has multiple lines.” Output: This is a test file.It has multiple lines. Explanation: The program reads and displays t 7 min read
- C program to delete a file The remove() function in C/C++ can be used to delete a file. The function returns 0 if the file is deleted successfully, Otherwise, it returns a non-zero value. The remove() is defined inside the <stdio.h> header file. Syntax of remove()remove("filename");ParametersThis function takes a string 2 min read
- C Program to merge contents of two files into a third file Let the given two files be file1.txt and file2.txt. The following are steps to merge. 1) Open file1.txt and file2.txt in read mode. 2) Open file3.txt in write mode. 3) Run a loop to one by one copy characters of file1.txt to file3.txt. 4) Run a loop to one by one copy characters of file2.txt to file 2 min read
- What is the difference between printf, sprintf and fprintf? printf: printf function is used to print character stream of data on stdout console. Syntax : printf(const char* str, ...); Example : C/C++ Code // simple print on stdout #include <stdio.h> int main() { printf("hello geeksquiz"); return 0; } Outputhello geeksquiz sprintf: String prin 2 min read
- Difference between getc(), getchar(), getch() and getche() All of these functions read a character from input and return an integer value. The integer is returned to accommodate a special value used to indicate failure. The value EOF is generally used for this purpose. getc() It reads a single character from a given input stream and returns the correspondin 2 min read
Miscellaneous
- time.h header file in C with Examples The time.h header file contains definitions of functions to get and manipulate date and time information. It describes three time-related data types. clock_t: clock_t represents the date as an integer which is a part of the calendar time. time_t: time_t represents the clock time as an integer which 4 min read
- Input-output system calls in C | Create, Open, Close, Read, Write System calls are the calls that a program makes to the system kernel to provide the services to which the program does not have direct access. For example, providing access to input and output devices such as monitors and keyboards. We can use various functions provided in the C Programming language 10 min read
- Signals in C language Prerequisite : Fork system call, Wait system call A signal is a software generated interrupt that is sent to a process by the OS because of when user press ctrl-c or another process tell something to this process. There are fix set of signals that can be sent to a process. signal are identified by i 5 min read
- Program error signals Signals in computers are a way of communication between the process and the OS. When a running program undergoes some serious error then the OS sends a signal to the process and the process further may not execute. Some processes may have a signal handler that does some important tasks before the pr 3 min read
- Socket Programming in C What is Socket Programming?Socket programming is a way of connecting two nodes on a network to communicate with each other. One socket(node) listens on a particular port at an IP, while the other socket reaches out to the other to form a connection. The server forms the listener socket while the cli 5 min read
- _Generics Keyword in C The _Generic keyword in C is used to implement a generic code that can execute statements based on the type of arguments provided. It can be implemented with C macros to imitate function overloading. The _Generic keyword was first introduced in C11 Standard. Syntax of _Generic in C_Generic( (express 3 min read
- Multithreading in C What is a Thread? A thread is a single sequence stream within a process. Because threads have some of the properties of processes, they are sometimes called lightweight processes. What are the differences between process and thread? Threads are not independent from each other unlike processes. As a 4 min read
- C Programming Interview Questions (2024) At Bell Labs, Dennis Ritchie developed the C programming language between 1971 and 1973. C is a mid-level structured-oriented programming and general-purpose programming. It is one of the oldest and most popular programming languages. There are many applications in which C programming language is us 15+ min read
- Commonly Asked C Programming Interview Questions | Set 1 What is the difference between declaration and definition of a variable/function Ans: Declaration of a variable/function simply declares that the variable/function exists somewhere in the program but the memory is not allocated for them. But the declaration of a variable/function serves an important 5 min read
- Commonly Asked C Programming Interview Questions | Set 2 This post is second set of Commonly Asked C Programming Interview Questions | Set 1What are main characteristics of C language? C is a procedural language. The main features of C language include low-level access to memory, simple set of keywords, and clean style. These features make it suitable for 3 min read
- Commonly Asked C Programming Interview Questions | Set 3 Q.1 Write down the smallest executable code? Ans. main is necessary for executing the code. Code is [GFGTABS] C void main() { } [/GFGTABS]Output Q.2 What are entry control and exit control loops? Ans. C support only 2 loops: Entry Control: This loop is categorized in 2 part a. while loop b. for loop 6 min read
- Top 50 C Coding Interview Questions and Answers (2024) C is the most popular programming language developed by Dennis Ritchie at the Bell Laboratories in 1972 to develop the UNIX operating systems. It is a general-purpose and procedural programming language. It is faster than the languages like Java and Python. C is the most used language in top compani 15+ min read
- C-Operators
- cpp-operator
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- C Programming Tutorial
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Function
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Cheat Sheet
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assignment Operators in C
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression.
The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol, which is defined as a simple assignment operator in C.
In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple Assignment Operator (=)
The = operator is one of the most frequently used operators in C. As per the ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed.
You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable, or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented Assignment Operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression "a += b" has the same effect of performing "a + b" first and then assigning the result back to the variable "a".
Run the code and check its output −
Similarly, the expression "a <<= b" has the same effect of performing "a << b" first and then assigning the result back to the variable "a".
Here is a C program that demonstrates the use of assignment operators in C −
When you compile and execute the above program, it will produce the following result −
- Career Schools - Jaipur - Jodhpur
- Apply as Mentor
01 Career Opportunities
02 beginner, 03 intermediate, 04 advanced, 05 training programs, c programming assignment operators, c programming free course with certificate: learn c in 21 days, what is an assignment operator in c, types of assignment operators in c, 1. simple assignment operator (=), example of simple assignment operator, 2. compound assignment operators, example of augmented arithmetic and assignment operators, example of augmented bitwise and assignment operators, practice problems on assignment operators in c, 1. what will the value of "x" be after the execution of the following code, 2. after executing the following code, what is the value of the number variable, benefits of using assignment operators, best practices and tips for using the assignment operator, about author, live batches schedule view all.

Assignment Operators In C | A Complete Guide With Detailed Examples
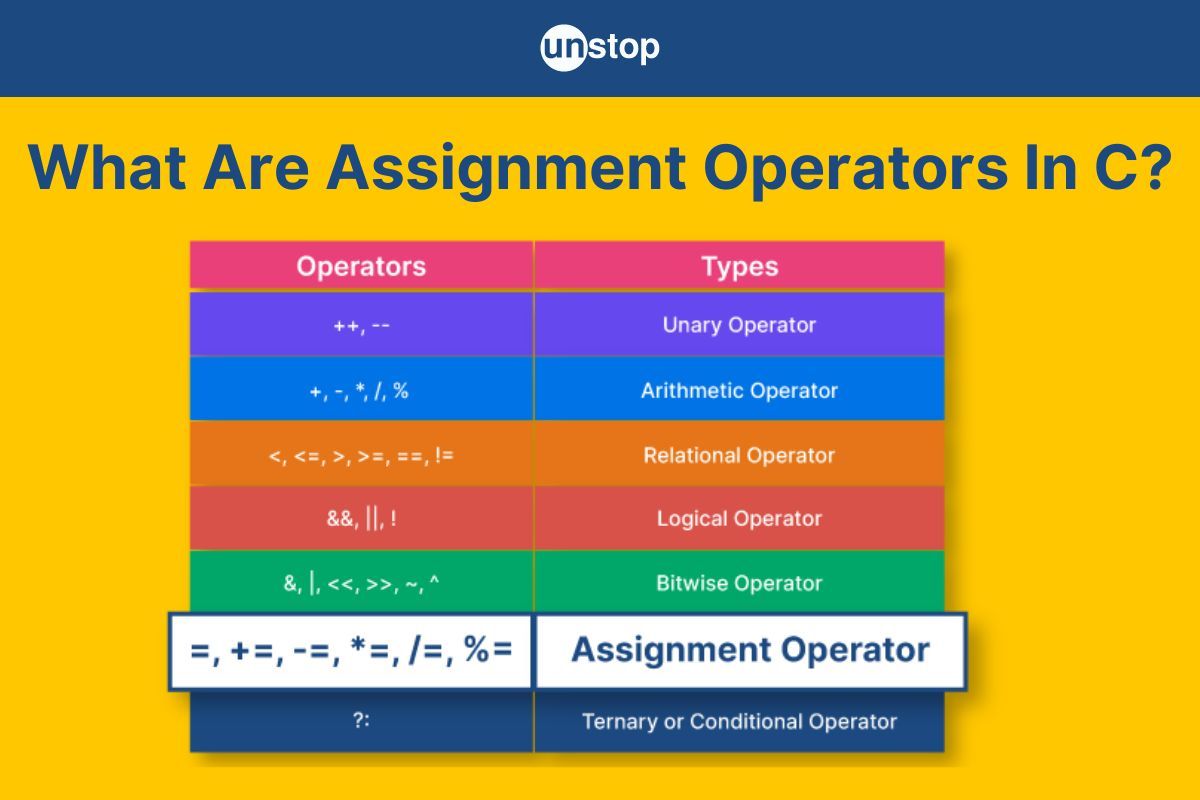
Table of content:
What are assignment operators in c, how assignment operators in c work step-by-step explanation, types of assignment operators in c, bitwise assignment operators in c.
- Precedence & Associativity Of Assignment Operators In C
Table Of Assignment Operators In C
Relational vs. assignment operators in c, frequently asked questions.
Operators are symbols or special characters that perform specific operations on one or more operands. They enable various computations and manipulation of data/ operands in C programs. These operands can be variables, constants, or expressions. It is important to understand the concept of various types of operators to utilize them effectively in writing efficient C code. In this article, we will focus on assignment operators in C.
Other types of operators include arithmetic, bitwise, logical, relational, ternary/ conditional, etc. Collectively, they enable complex computations, comparisons, and control flow within programs, allowing developers to manipulate data in a variety of ways.
In simple terms, assignment operators in C programming are used to assign values to variables. This includes assigning initial value as well as updating the value of a variable with a new value based on a certain operation or calculation. The most commonly used assignment operator is the equal-to operator (=), which assigns the right-hand side value to the variable on the left-hand side of the operator.
For example, the expression x = 5 assigns the value of 5 to the variable x.
Other assignment operators in C include addition assignment (+=), subtraction assignment (-=), multiplication assignment (*=), division assignment (/=), and modulus/ modulo assignment (%=). They are also referred to as compound assignment operators. As their name suggests, they are used to assign the resultant value of addition, subtraction, multiplication, division, and modulus operations (respectively) to the left-side variable/ operand.
For example, the equation x += 5 is equivalent to x = x + 5. It updates the value of x by adding 5 to its current value.
These assignment operators can be combined with other operators to perform more complex operations in a single statement. For example, x += y * 3 updates the value of x by adding three times the value of y to its current value.
Using basic assignment operators can help make code more concise and easier to read and improve performance by reducing the number of operations required. However, it is important to use them carefully and avoid unintended consequences, such as side effects or errors. We will discuss each of these assignment operators in detail ahead, but first let's look at the basic syntax for this.
Syntax For Assignment Operators In C:
variable_name operator expression;
Here,
- The variable_name refers to the name/ identifier of the variable to whom you are assigning (or updating) a value.
- The term operator refers to the respective assignment operator you are using.
- Expression is the right-hand operand, i.e., it refers to the value you assign to the variable.
Here are some examples of assignment operators and their syntax:
x = 5; // assigns the value of 5 to the variable x y += 10; // adds 10 to the current value of y and updates y with the result z *= 3; // multiplies the current value of z by 3 and updates z with the result
Given below is a stepwise description of the working mechanism of assignment operators in C.
- Step 1 (The variable): The first step is to select the variable that we want to assign a value to. This variable can be of any data type, such as integer, float, or char .
- Step 2 (The operator): The second step is to select the assignment operator that we want to use. As mentioned, the most commonly used assignment operator is the simple assignment operator (=), to assign the value of the right side operand to the left side operand.
- Step 3 (The value or expression): The third step is to provide the value (or expression for calculating value) we want to assign to the variable. This value can be a constant or an expression that combines variables and constants using arithmetic or logical operators .
- Step 4 (The evaluation): After that, the compiler evaluates the expression on the right-hand side of the operator. This evaluation involves performing any necessary logical or arithmetic operations based on the precedence rules of the operators.
- Step 5 (The assignment): Finally, it assigns the evaluated value to the variable on the left-hand side of the assignment operator. If we are updating the value, this assignment replaces the previous value of the variable with the new value.
Let's look at a basic C program example illustrating both these approaches of using assignment operators.
Code Example:
The initial values of x and y are: 5, 10 The updated value of x is: 15
Explanation:
We begin the simple C program example by including the <stdio.h> header file for input/output operations.
- We then initiate the main() function , which is the entry point of the program's execution.
- Inside main(), we declare two integer variables , x and y, and assign the values of 5 to x and 10 to y. Then, we print these initial values to the console using the printf() function .
- Next, we use the addition assignment operator (as mentioned in the code comments ) to add the initial value of y to the initial value of x and assign the result to x.
- The operation involves adding the value of y, which is 5, to the value of x, which is 10. The output is, hence, 15.
- Once again, we use the printf() function to display the updated value of x.
All the assignment operators in C programming language can be classified into two major types with subtypes. These types and the operators included in them are mentioned below-
Simple Assignment Operator In C (=)
There is only one assignment operator that falls into this category. This is the simplest assignment operator whose only purpose is to assign the value given on the right to the variable on the left. The basic assignment operator symbol is a single equals sign (=). It is the most commonly used assignment operator in C language. Below is an example of how to use this operator in simple assignment expressions.
The value of x is 5
In the simple C code example , we include the standard input-output header file, <stdio.h>, which contains the library functions for input and output operations in C programming.
- Inside main() funtion , we declare an integer variable named x and initialize it with the value 5 using the simple assignment operator .
- This step reserves a memory location to store an integer value and assigns the value 5 to x.
- Next, we use the printf() function to display the output with a descriptive string message (using formatted string) .
- Here, we use the %d format specifier as a placeholder for the integer value and the newline escape sequence (\n) to shift the cursor to the next line afterwards.
- Lastly, the program successfully completes execution with the return 0 statement . It indicates the successful termination of the main() function and the program as a whole.
It will be easier to understand why we call this operator simple when you check out the other category, i.e., compound operators.
Compound Assignment Operators In C
The compound assignment operators are called as such because they combine a binary operation with the assignment operation. They perform a binary operation on the values of the variable and the value on the right-hand side and then assign the result to the variable on the left-hand side/ left operand.
There are five types of compound assignment operator in C:
- Subtraction Assignment Operator (-=)
- Addition Assignment Operator (+=)
- Multiplication Assignment Operator (=)
- Division Assignment Operator (/=)
- Modulus Assignment Operator (%=)
The advantage of using these operators is that they provide a shorthand notation for performing common operations such as addition, multiplication, division, etc.
Now that you have a broad idea of what compound assignment operators in C are, let's explore each type of assignment operator in detail.
Addition Assignment Operator In C (+=)
The addition assignment operator adds the value given on its right side to the current value of the variable on the left. It then assigns the resultant value back to the variable on the left.
In other words, it adds the initial value of the first variable to the initial value of a second variable and assigns it back to the first variable. Below is a C program example illustrating the use of this compound assignment operator.
The value of x is 8
In the C code example, we first include the essential header files.
- Inside the main() function , we declare an integer variable, x , and initialize it with the value 5 using the simple assignment operator.
- Next, we use the addition assignment operator (+=) to add a value 3 to x (variable on the left side) and assign the result back to it.
- Here, we add 3 to x's initial value and reassign it back. That is, x += 3 is equivalent to x = x + 3, which adds 3 to x's value and stores the result back into x.
- Then, we use the printf() function to display the output to the console, after which the program terminates with the return 0 statement.
Check out this amazing course to become the best version of the C programmer you can be.
Subtraction Assignment Operator In C (-=)
The subtraction assignment operator is used to subtract a given value, mentioned on the right side of the operator, from the initial value of the variable on the left (i.e., the left operand). It then assigns the result back to the original variable on the left. Alternatively, we can also subtract the value of a second variable from the initial variable.
The value of x is 7
In the example C program -
- Inside the main() function , we declare an integer variable x and assign the value 10 to it using the simple operator.
- We then use the subtraction assignment operator (-=) to subtract a value 3 from the initial value of x. The result is assigned back to x using this operator.
- Here, the expression x -= 3 is equivalent to x = x - 3, which means we subtract 3 from 5 (i.e., the value of x), and the value of x is updated with the result.
- After that, we use the printf() function to display the updated value of the variable x to the console.
Multiplication Assignment Operator In C (*=)
The multiplication assignment operator in C (*=) first multiplies a given value (on the right) with the initial/ current value of the variable on the left (left operand). Then, it assigns the result back to the variable on the left. Alternatively, it can also be used to multiply the initial value of a variable by the value of another variable in the program.
The value of x is 20
In the example C code -
- Inside the main() function , we declare an integer variable named x and initialize it with the value 5 using the simple assignment operator.
- Next, we use the multiplication assignment operator to multiply the initial value of x by 4 and then assign the result back to variable x.
- That is, the expression x *= 4 implies x = x * 4, which multiplies the value of x by 4 and stores the result back into x.
- Using the printf() function , we display the updated value of x and a string literal message.
Division Assignment Operator In C (/=)
The division assignment operator (/=) first divides the current/ initial value of the variable mentioned on its left side by the value given on its right. Then, the revised value/ result is assigned back to the variable on the left. Alternatively, we can also use this operator to divide the value of one variable by another variable and then update the value of one.
Let's take a look at an example that illustrates the use of this compound assignment operator in C code.
In the sample C program -
- We declare an integer variable x inside the main() function and initialize it with the value 10.
- Next, we use the division assignment operator (/=) to divide the value of x by 2 and assign the result of this operation back to variable x.
- Here, the expression x /= 2 can be expanded as x = x / 2, which ultimately divides the value of x by 2 and stores the result back into x.
- Finally, we display this output using the printf() function with a formatted string message.
Modulo Assignment Operator In C (%=)
The modulo assignment operator (%=) is a combination of the modulo arithmetic operator and assignment operator. It divides the value of the left-side operand by the right-side operand to calculate the remainder of this division. Then, it assigns the resulting remainder back to the variable on the left. Note that the value on the right can also be the value of another variable in the program.
Look at the example below to better understand how to apply this compound assignment operator in C programs.
The value of x is 3
In the sample C code -
- In the main() function , we declare a variable, x, of integer data type and initialize it with the value of 14.
- Then, we use the modulo assignment operator ( %=) to calculate the remainder from the division of the variable on the left side by a specified value and assign the result back to that variable.
- In this example, we divide the value of x by 4 and reassign the remainder to it.
- That is, x %= 4 is equivalent to x = x % 4, which ultimately calculates the remainder when x is divided by 4 and stores the result back into x.
- This reassigned/ updated value of x is displayed on the console using the printf() function with a formatted string and %d format specifier.
We have already discussed the most commonly used assignment operators in C programing language. There are a few other compound assignment operators that you must know about. These are the bitwise assignment operators, which first conduct the respective bitwise operation on the operands and then assign the result back to the left operand in the expression.
There are three types of bitwise assignment operators in C, which we have discussed ahead.
Bitwise AND Assignment Operator In C (&=)
The bitwise AND assignment operator (&=) performs a bitwise AND operation between the current value of the variable on the left (i.e., the left operand) and the value on the right (i.e., the right operand) and then assigns the result to the variable on the left. The value on the right can also be another variable.
The assignment operator example below showcases how this compound operator works.
The value of x is 4
In the C program sample , we define the main() function (which serves as the entry point) after including the <stdio.h> file.
- We then declare an integer variable called x and use the basic assignment operator to initialize it with the value 12.
- Next, we use the bitwise AND assignment operator (&=) to perform a bitwise AND operation between the value of the variable on the left side and a specified value, and then we assign the result back to that variable.
- The equation x &= 5 , which implies x = x & 5, performs a bitwise AND between the value of x and 5 and stores the result back into x.
- We display the result of this operation to the console with the printf() function , a formatted string, and the %d specifier.
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
Bitwise OR Assignment Operator In C (|=)
The bitwise OR assignment operator (|=) performs a bitwise OR operation between the current value of the variable on the left and the value on the right and then assigns the result to the variable on the left. The example below showcases the use this compound assingment operator in C.
Output Window :
The value of x is 13
Code Explanation:
In the C code sample -
- In the main() function , we declare an integer variable x and initialize it with the value 12. This reserves a memory location to store an integer value.
- Next, using the bitwise OR assignment operator , we perform a bitwise OR operation between the value of the variable on the left side (i.e., 12) and a specified value (i.e., 5) and assign the result back to the left-side variable, i.e., x.
- The expression x |= 5 leads to the evaluation of x = x | 5, which performs a bitwise OR between the value of x and 5 and stores the result back into x.
- Then, we use the printf() function to display the result/ updated value of left operand x to the console. We also use a formatted string and the %d specifier inside printf().
Left Shift Assignment Operator In C (<<=)
The bitwise left shift assignment operator (<<=) first shifts the bits of the current value of the variable on the left (i.e., left-hand operand) by the number of positions specified by the value on the right. Then, it assigns the result of this operation back to the variable on the left.
The value of x is 40
In this assignment operator example, we first include the essential header file and then initiate the main() function .
- Inside main(), we declare an integer variable x and initialize it with the value 10. This step reserves a memory location to store an integer value and assigns the value 10 to x.
- Next, we apply the left shift assignment operator (<<=) on this variable to perform a left shift operation on its value by a specified number of bits (here, 2) and assign the result back to that variable.
- The equation x <<= 2 expands to x = x << 2, which performs a left shift on the value of x by 2 bits and stores the result back into x.
- We print the new value of the left-hand side variable x to the console using the printf() function .
Right Shift Assignment Operator In C (>>=)
The bitwise right shift assignment operator (>>=) begins by shifting the bits of the initial value of the variable on the left (i.e., left-hand operand) by the number of positions specified by the right-hand value and then assigns the result to the variable on the left.
In the assignment operator C example-
- Inside the main() function (which serves as the entry point for program execution), we declare an integer variable x and initialize it with the value 10.
- Next, we employ the right shift assignment operator ( >>=) to shift the bits of the value on the left side (i.e., 10, the value of x) by a specified number of positions (i.e., 1) to the right. Then, assign the result back to the left-hand side variable.
- The equation x >>= 1 is equivalent to x = x >> 1, which performs a right shift operation on the value of x by 1 position and stores the result back into x.
- We publish this updated value of the variable x to the console using a formatted string and %d specifier inside the printf() function .
Also Read: Shift Operators In C | The Ultimate Guide With Code Examples
Precedence & Associativity Of Assignment Operators In C
In C, operators have a defined precedence that determines the order in which expressions are evaluated when multiple operators are present. Precedence helps in resolving which operator should be applied or evaluated first in an expression. Associativity, on the other hand, defines the direction in which operators of the same precedence level are processed.
Precedence of Assignment Operators In C
All assignment operators in C (=, +=, -=, *=, /=, %=, etc.) have lower precedence compared to most other operators, such as arithmetic operators (+, -, *, /), relational operators (<, >, <=, >=) , and logical operators (&&, ||). This means that in an expression where assignment operators are used alongside other operators, the other operators are evaluated first before the assignment takes place.
In other words, the other operators take precedence over assignment operators when evaluating expressions in C programs. For example:
int a = 5; int b = 10; int c = a + b * 2;
In this expression, the multiplication operator (*) has higher precedence than the addition operator (+), and both have higher precedence than the assignment (=). Therefore, b * 2 is evaluated first, then the result is added to a, and finally, the sum is assigned to c.
Assignment Operator Associativity In C
Assignment operators in C have right-to-left associativity. This means that when multiple assignment operators are used in a single expression, the expression is evaluated from right to left.
int a, b, c; a = b = c = 10;
In this case, the value 10 is first assigned to c, then the value of c is assigned to b, and finally, the value of b is assigned to a. Since every assignment operator associates from right to left, this evaluation happens from right to left.
This associativity of assignment operators in C is particularly important to understand when chaining assignments or combining them with other operations.
Here is a table listing all the assignment operators in C programming with a brief description and example.
Both the relational and the assignment operators serve different purposes and have distinct characteristics in programming languages like C. Assignment operators deal with assigning values to operands/ variables. In contrast, relational operators focus on making comparisons between operands, thus enabling logical decision-making in control flow statements .
Here are some points highlighting the differences between relational and assignment operators in C:

Purpose | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators are used to assign values or expressions to variables. They perform the task of storing a value in a variable.
Relational Operators: Relational operators are used to compare values or expressions. They determine the relationship between two operands and produce a Boolean result (true or false) based on the comparison.
Syntax | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators are typically represented by a single character followed by an equal sign, such as (=), (+=), (-=), (*=), etc.
Relational Operators: Relational operators are represented by two or more characters, including equality operators (==, !=), other relational operators (<, >, <=, >=), etc.
Operation | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators in C perform the task of assigning a value or expression to a variable. They modify the value of the left-hand side variable based on the right-hand side value or expression.
Relational Operators: Relational operators compare the values of two operands and return a Boolean result indicating whether the comparison is true or false.
Result Type | Relational Vs. Assignment Operators In C
Assignment Operators: In case of the simple assignment operator, the result is not a value in itself but the assignment of value to the variable on the left-hand side of the operator. But in case of compound assingment operators in C the result is the value assigned to the variable on the left-hand side of the operator, which can be used in further expressions or assignments.
Relational Operators: The result of a relational operator is a Boolean value, either true or false, indicating the outcome of the comparison.
Usage | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators in C are primarily used for variable assignments, updating variables, and performing calculations involving the current value of a variable.
Relational Operators: Relational operators are used in conditional statements (if, while, for) and expressions that require comparison between variables or values.
Precedence | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators in C have lower precedence compared to most other operators, which means they are evaluated after most other operations in an expression.
Relational Operators : Relational operators have higher precedence than assignment operators, allowing them to be evaluated before assignment operations.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here .
The assignment operator in C is one of the most important and commonly used operators in the language. It assigns values to variables, which is essential for writing any program. Compound assignment operators can be used to make code more concise and efficient. These assignment operators are fundamental operators that allow programmers to store and manipulate data effectively throughout their programs. It is important to understand the different types of assignment operators and how to use them correctly to write and run efficient and effective C code .
Also Read: 100+ Top C Interview Questions With Answers (2024)
Q. What is an assignment operator in C?
An assignment operator is an operator in the C language that assigns a value to a variable. This could be a direct value (as in initialization) or the value that results from a compound operation (like the result of addition, subtraction, multiplication, etc. operations).
The most commonly used assignment operator is the equal sign (=), the basic assignment operator. It assigns the value on the right-hand side to the variable on the left-hand side of the operator.
Q. What is the difference between a simple assignment operator and a compound assignment operator?
The simple assignment operator, the equal-to operator (=), assigns a single value to a variable. On the other hand, a compound assignment operator in C (binary operator) performs a binary operation (such as addition, subtraction, multiplication, etc.) between the current value of a variable and another value or expression and then assigns the result to the variable. Its examples are- addition assignment (+=), multiplication assignment (*=), modulo assignment (%=), etc.
Q. What happens if I use an assignment operator with incompatible types in C?
Using an assignment operator with incompatible types in the C programming language results in a compilation error . C is a statically typed language, meaning variables must be explicitly declared with their data types, and the compiler enforces strict type-checking during the compilation process . If an attempt is made to assign a value of one type to a variable of a different, incompatible type without an explicit type conversion , the compiler will raise an error.
For example, trying to assign a character ('A') to an integer variable declared as int will lead to a compilation error. This mechanism helps catch potential type-related errors early in the development process, ensuring that the program adheres to the specified type rules and preventing unexpected behavior during runtime.
Q. Can I chain multiple assignment operators together in C language?
In the C programming language, it is possible to chain multiple assignment operators together in a single statement. That is, you can assign the same value to multiple variables in one line by chaining assignments. For example, the following code is valid in C:
int a, b, c; a = b = c = 5; // This is not allowed in C
This assigns the value 5 to all three variables (a, b, and c). The expression is evaluated from right to left due to the right-associative nature of the assignment operators in C.
Q. Are there any precedence rules for assignment operators?
Yes, there are precedence rules for assignment operators in C language. The assignment operator (=) has a lower precedence than most other operators. This means that when an expression contains both assignment and other operators, the assignment will be performed after the evaluation of the other operators.
For example:
int a, b, c; a = b = c = 5 + 3 * 2;
In this example, the multiplication (*) has higher precedence than the assignment (=). So, 3 * 2 is evaluated first, resulting in 6. Then, 5 + 6 is evaluated, resulting in 11. Finally, the assignment is performed from right to left, so c, b, and a will all be assigned the value 11.
It's important to note that associativity also plays a role. The assignment operators in C are right-associative, which means that when multiple assignments appear in a row, they are evaluated from right to left. In the example above, c = 5 + 3 * 2 is evaluated first, followed by b = c, and finally a = b.
Now that you know all about assignment operators in C, check out some other interesting topics:
- Increment And Decrement Operators In C With Precedence (+Examples)
- Keywords In C Language | Properties, Usage, Examples & Detailed Explanation
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Ternary (Conditional) Operator In C Explained With Code Examples
- For Loop In C | Structure, Working & Variations With Code Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
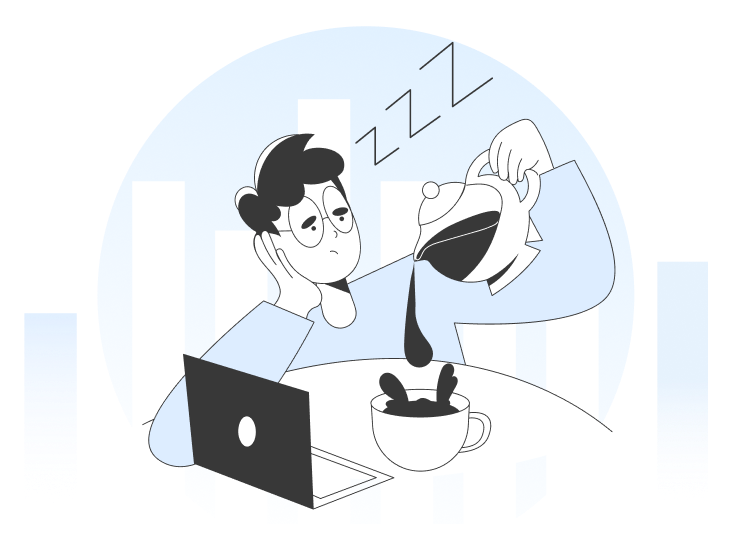
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

to our newsletter
Blogs you need to hog!
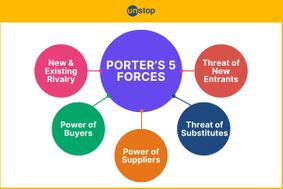
Porter's 5 Forces: Comprehensive Guide To This Analytical Tool
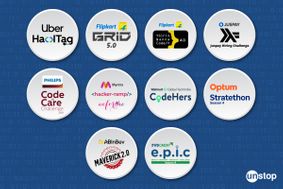
How To Organize Hackathons & Coding Competitions
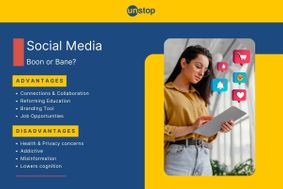
Social Media: Boon Or Bane? Delving Deep Into The Debate
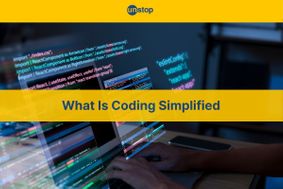
What Is Coding | Role, Working, How To Learn & More Simplified
Home » Learn C Programming from Scratch » C Assignment Operators
C Assignment Operators
Summary : in this tutorial, you’ll learn about the C assignment operators and how to use them effectively.
Introduction to the C assignment operators
An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable:
After the assignmment, the counter variable holds the number 1.
The following example adds 1 to the counter and assign the result to the counter:
The = assignment operator is called a simple assignment operator. It assigns the value of the left operand to the right operand.
Besides the simple assignment operator, C supports compound assignment operators. A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
The following example uses a compound-assignment operator (+=):
The expression:
is equivalent to the following expression:
The following table illustrates the compound-assignment operators in C:
- A simple assignment operator assigns the value of the left operand to the right operand.
- A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
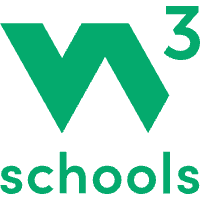
C Programming Tutorial
Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
Functions in C
- C - Functions
- C - Main Function
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
Arrays in C
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Header Files
Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
Assignment Operators in C
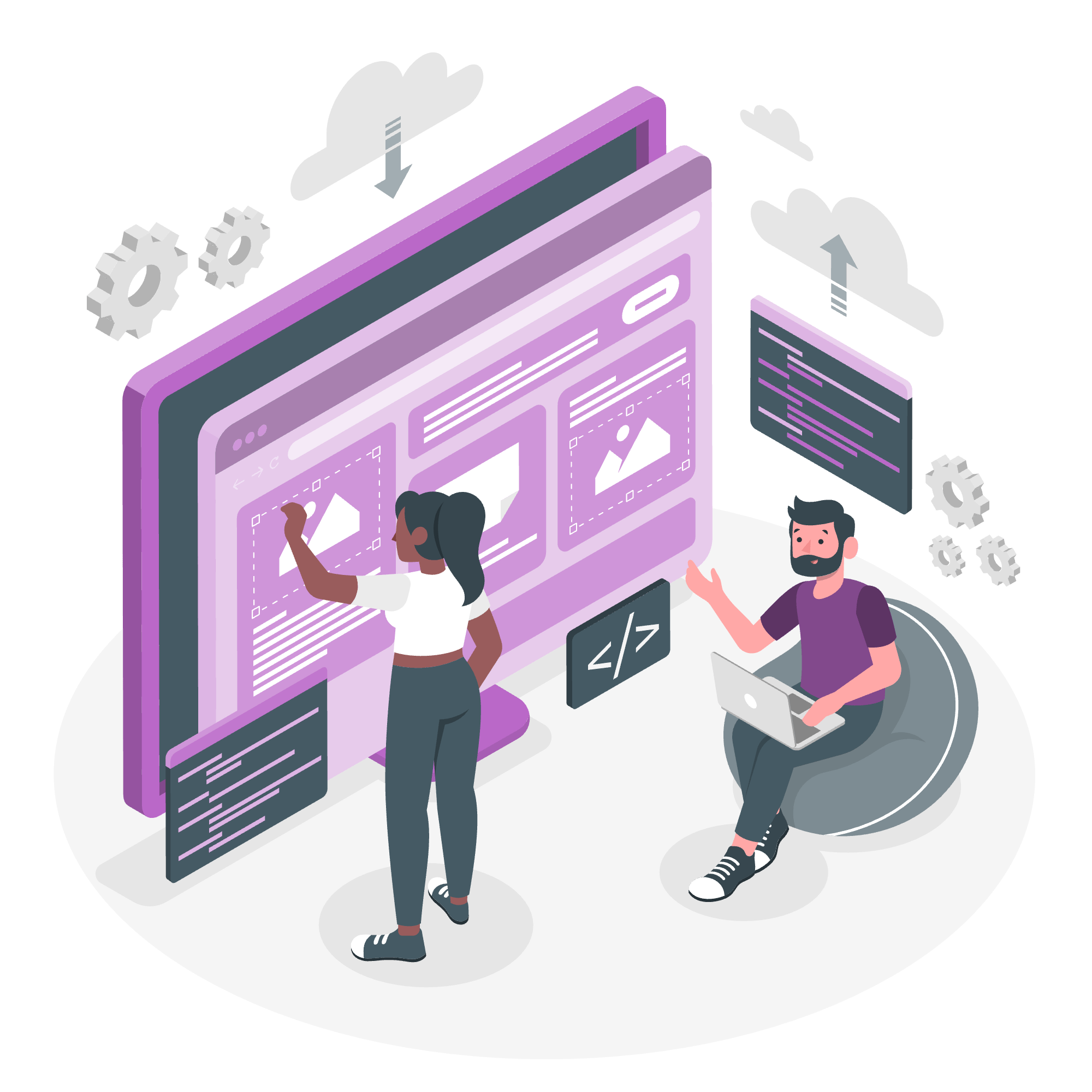
What Are Assignment Operators?
Before we jump in, let's understand what assignment operators are. Think of them as the equals sign (=) you used in math class, but with superpowers! They're used to assign values to variables in your programs. But in C, they can do much more than just simple assignments.
Simple Assignment Operator (=)
Let's start with the basics – the simple assignment operator. It's the foundation of all assignment operations in C.
How It Works
The simple assignment operator is represented by the equals sign (=). It takes the value on the right side and assigns it to the variable on the left side.
Here's a simple example:
In this code, we're declaring an integer variable called age and then assigning it the value 25. It's as simple as that!
Multiple Assignments
You can also chain assignments together. Let's look at an example:
This code assigns the value 10 to all three variables: x, y, and z. It works from right to left, so first z gets 10, then y gets the value of z (which is 10), and finally x gets the value of y (which is also 10).
Initializing Variables
You can also use the assignment operator when you're declaring variables:
This is a great way to set initial values for your variables right when you create them.
Augmented Assignment Operators
Now, let's level up! Augmented assignment operators are like shortcuts. They perform an operation and an assignment in one step. Let's look at them one by one:
Addition Assignment (+=)
The += operator adds the right operand to the left operand and assigns the result to the left operand.
Subtraction Assignment (-=)
The -= operator subtracts the right operand from the left operand and assigns the result to the left operand.
Multiplication Assignment (*=)
The *= operator multiplies the left operand by the right operand and assigns the result to the left operand.
Division Assignment (/=)
The /= operator divides the left operand by the right operand and assigns the result to the left operand.
Modulus Assignment (%=)
The %= operator calculates the remainder when the left operand is divided by the right operand and assigns the result to the left operand.
Bitwise AND Assignment (&=)
The &= operator performs a bitwise AND operation and assigns the result to the left operand.
Bitwise OR Assignment (|=)
The |= operator performs a bitwise OR operation and assigns the result to the left operand.
Bitwise XOR Assignment (^=)
The ^= operator performs a bitwise XOR operation and assigns the result to the left operand.
Left Shift Assignment (<<=)
The <<= operator performs a left shift operation and assigns the result to the left operand.
Right Shift Assignment (>>=)
The >>= operator performs a right shift operation and assigns the result to the left operand.
Summary Table of Assignment Operators
Here's a handy table summarizing all the assignment operators we've covered:
And there you have it! You've just taken a grand tour of assignment operators in C. Remember, practice makes perfect. Try writing some code using these operators, and you'll soon find yourself using them effortlessly.
Happy coding, future C programmers!
Credits: Image by storyset

IMAGES
VIDEO
COMMENTS
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators.This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left.
Assignment Operators in C Programming. Overview. In C programming, assignment operators are used to assign values to variables. The simple assignment operator is =. ... Example: Here the simple assignment operator = is used to assign a value to a variable. Code: #include <stdio.h> int main() { int a = 5; // Prefix increment: 'a' is incremented ...
Assignment Operators in C - In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression. ... Example 3. Here is a C program that demonstrates the use of assignment operators in C − ...
The left side of an assignment operator is a variable and on the right side, there is a value, variable, or an expression. It computes the outcome of the right side and assign the output to the variable present on the left side. C supports following Assignment operators: 1. Simple Assignment = Operator Example
Learn all about Assignment Operators in C with detailed explanations, examples, and types. A complete guide to understanding their usage in C programming.
The Assignment operators in C are some of the Programming operators that are useful for assigning the values to the declared variables. Equals (=) operator is the most commonly used assignment operator. For example: int i = 10; The below table displays all the assignment operators present in C Programming with an example.
Assignment Operators in C are used to assign values to the variables. The left side operand is called a variable and the right side operand is the value. The value on the right side of the "=" is assigned to the variable on the left side of "=". In this C tutorial, we'll understand the types of C programming assignment operators with examples.
Output: The value of x is 8. Explanation: In the C code example, we first include the essential header files.. Inside the main() function, we declare an integer variable, x, and initialize it with the value 5 using the simple assignment operator.; Next, we use the addition assignment operator (+=) to add a value 3 to x (variable on the left side) and assign the result back to it.
Summary: in this tutorial, you'll learn about the C assignment operators and how to use them effectively. Introduction to the C assignment operators. An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable:
Assignment Operators in C. Hello there, future programmers! Today, we're going to dive into the wonderful world of assignment operators in C. Don't worry if you've never written a line of code before - I'm here to guide you through this journey step by step. By the end of this tutorial, you'll be assigning values like a pro!