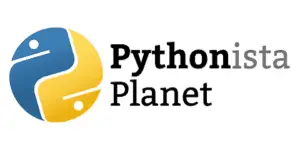

18 Python while Loop Examples and Exercises
In Python programming, we use while loops to do a task a certain number of times repeatedly. The while loop checks a condition and executes the task as long as that condition is satisfied. The loop will stop its execution once the condition becomes not satisfied.
The syntax of a while loop is as follows:
In this post, I have added some simple examples of using while loops in Python for various needs. Check out these examples to get a clear idea of how while loops work in Python. Let’s dive right in.
1. Example of using while loops in Python
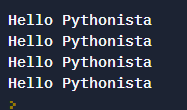
2. Example of using the break statement in while loops
In Python, we can use the break statement to end a while loop prematurely.
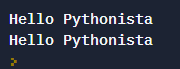
3. Example of using the continue statement in while loops
In Python, we can use the continue statement to stop the current iteration of the while loop and continue with the next one.
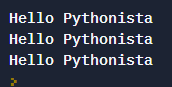
4. Using if-elif-else statements inside while loop
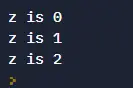
5. Adding elements to a list using while loop
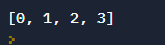
6. Python while loop to print a number series

7. Printing the items in a tuple using while loop
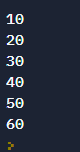
8. Finding the sum of numbers in a list using while loop
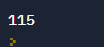
9. Popping out elements from a list using while loop
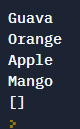
10. Printing all letters except some using Python while loop
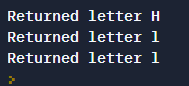
11. Python while loop to take inputs from the user
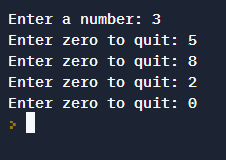
12. Converting numbers from decimal to binary using while loop
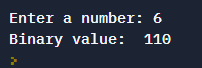
13. Finding the average of 5 numbers using while loop
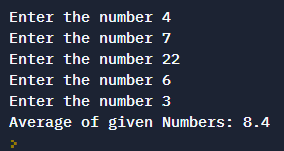
14. Printing the square of numbers using while loop
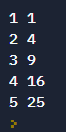

15. Finding the multiples of a number using while loop
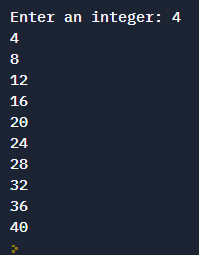
16. Reversing a number using while loop in Python
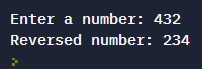
17. Finding the sum of even numbers using while loop
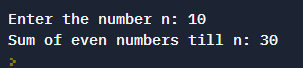
18. Finding the factorial of a given number using while loop
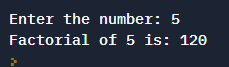
I hope this article was helpful. Check out my post on 21 Python for Loop Examples .
I'm the face behind Pythonista Planet. I learned my first programming language back in 2015. Ever since then, I've been learning programming and immersing myself in technology. On this site, I share everything that I've learned about computer programming.
17 thoughts on “ 18 Python while Loop Examples and Exercises ”
I am looking for a way to take user inputs in while loop & compare it & print the smallest/largest value. Can you help?
9.) Popping out elements from a list using while loop thank your us this kind of content for free appreciate 🙂 i was curious this # 9.)
fruitsList = [“Mango”,”Apple”,”Orange”,”Guava”]
while len(fruitsList) > 3: fruitsList.pop() print(fruitsList)
n=”e” numlist = [] while n != “” n= int(input (“Enter A Number: “)) numlist.append(n) print(numlist) print(max(numlist)) print (min(numlist))
Write a program that reads a value V, and then starts to read further values and adds them to a List until the initial value V is added again. Don’t add the first V and last V to the list. Print the list to the console.
Input Format
N numbers of lines input, with a random string
Output Format
Single line output, as a list.
Sample Input 1
56 23 346 457 234 436 689 68 80 25 567 56
Sample Output 1
[’23’, ‘346’, ‘457’, ‘234’, ‘436’, ‘689’, ’68’, ’80’, ’25’, ‘567’]
x = int(input(‘How many users will actually provide numerical values?’)) i = x internal_list = []
def main(List:list!=None):
print(f”The given list of numerical entities is formed by {internal_list}”) if i >= x: print(f”The maximal value contained by user’ list is equal with… {max(internal_list)}”) else: pass
x = 1 while i >= x: j: int = input(“Could you introduce your personal number?”) internal_list.append(j) x += 1 if i<=x: main(List=internal_list) break
You can append the numbers in the list and find the minimum or maximum.
i=0 newlist=[] #create an empty list while i<5: #for 5 values x=int(input(' Enter numbers')) i+=1 newlist.append(x) print(newlist) #you may skip this line print("The smallest number is", min(newlist))
The output will be: Enter numbers 3 Enter numbers 4 Enter numbers 8 Enter numbers 2 Enter numbers 9 [3,4,8,2,9] The smallest number is 2
My bro ,u are too much oo,u really open my eyes to many things about pythons ,which I did not know b4 .pls am a beginer to this course .I need yr help oo,to enable me know more sir. Thanks and God bless u
Printing the items in a tuple using while loop exercise shows wrong answer please review the answer.
I just checked again, and it is the correct answer. Can you check your code once again? Maybe you might have missed something in your code.
Hi, Ashwin Thanks for these informative and variative while loops examples; they are really helpful for practicing. 🙂
name = input(‘name’) strip= input(‘word’)
# Now i want to see both methods while and for to striped out the strip’s values from the name’s letters.
no output is coming in example 10.
lst = [] for i in range(5): num = int(input(“Enter your numbers: “)) lst += [num] print(“The greater number is”,max(lst)) print(“The smallest number is”,min(lst))
How I wish you were able to write comments on your code lines to help newbies better understand the concept.
Rony its true but what do you mean by write coments on your code
great im loving this exercises.i feel on top of the world
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name and email in this browser for the next time I comment.
Recent Posts
Introduction to Modular Programming with Flask
Modular programming is a software design technique that emphasizes separating the functionality of a program into independent, interchangeable modules. In this tutorial, let's understand what modular...
Introduction to ORM with Flask-SQLAlchemy
While Flask provides the essentials to get a web application up and running, it doesn't force anything upon the developer. This means that many features aren't included in the core framework....
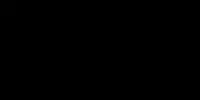
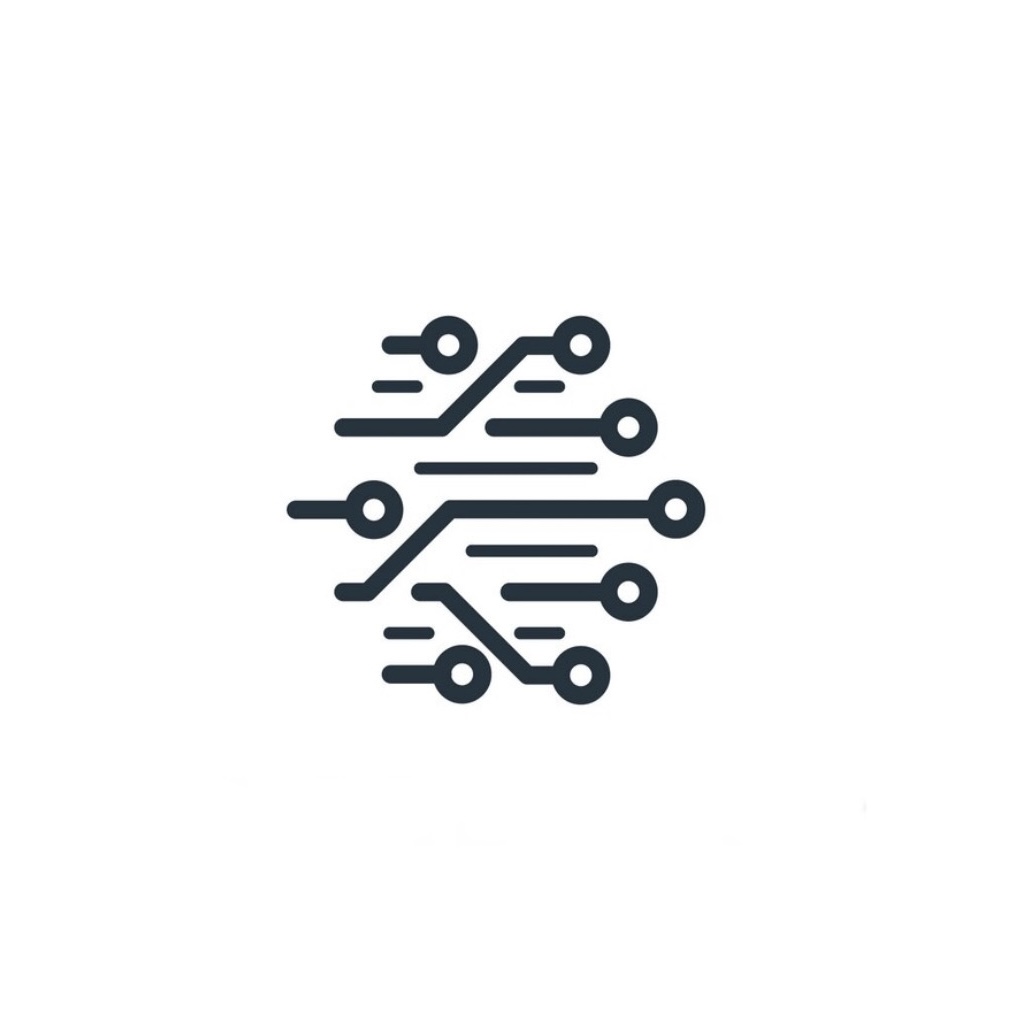
Python: Assigning Values to Variables During While Loop Conditions
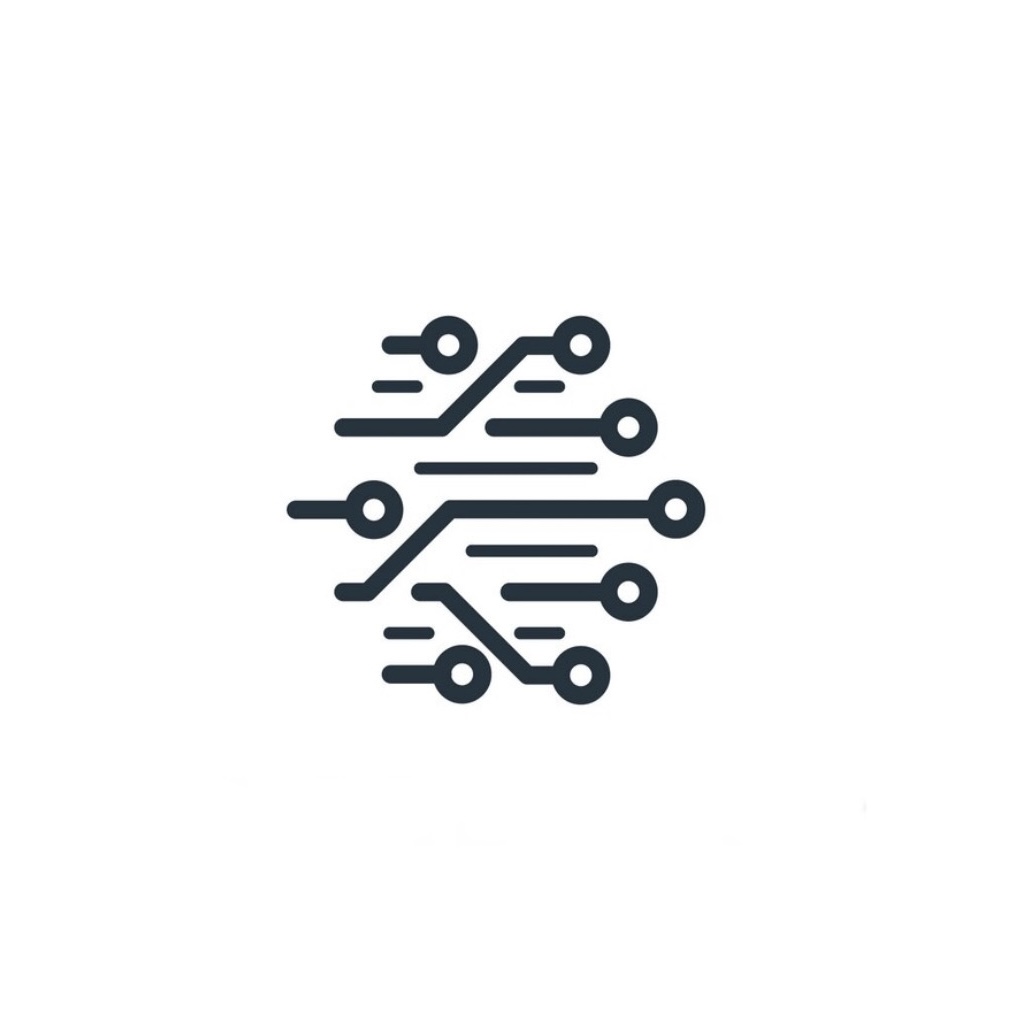
Python's while loop is a powerful tool for iterating through code blocks as long as a certain condition remains true. You can further enhance this functionality by assigning values to variables directly within the loop's condition. This approach can significantly streamline your code, making it more efficient and elegant.
Understanding the Basics of While Loops
Before diving into the nuances of variable assignment during loop conditions, let's review the fundamental structure of a while loop. In Python, a while loop executes a block of code repeatedly as long as a specified condition evaluates to True .
The condition can be any expression that evaluates to either True or False . For instance, you might have a condition like x < 10 or user_input != "quit" . Once the condition becomes False , the loop terminates, and the program execution continues from the line after the loop.
Assigning Values Within Loop Conditions
The core concept lies in utilizing the and and or logical operators within the loop condition to simultaneously assign values to variables and evaluate the condition. Let's illustrate this with a simple example:
In this example, we initialize a counter variable and use it in the loop condition ( counter < 5 ). The loop continues as long as counter is less than 5. Within the loop, we increment counter by 1 after each iteration.
Now, let's consider a scenario where we want to read user input repeatedly until a specific string is entered. We can achieve this elegantly by assigning the user input to a variable within the loop condition:
Here, the loop continues as long as the user input is not equal to "quit". During each iteration, we capture the user input using input() and assign it to the user_input variable. If the user enters "quit", the condition becomes False , and the loop terminates.
Advantages of Assigning Values in While Loop Conditions
Conciseness: By assigning values within the loop condition, you eliminate the need for separate assignment statements before or within the loop body, resulting in cleaner and more compact code.
Clarity: This approach often improves code readability by directly relating the condition being evaluated to the variable assignments. It emphasizes the connection between the loop's continuation and the variables involved.
Efficiency: In certain cases, this method can offer performance improvements by reducing the number of lines of code executed. For example, if you're working with a large dataset, you might save time by avoiding unnecessary variable assignments.
Practical Applications
Let's explore some practical scenarios where assigning values within loop conditions shines:
1. User Input Validation: You can use this technique to repeatedly prompt the user for input until they provide valid data. For example, you could request a number within a specific range:
2. Processing Data in Batches: You can process large datasets in smaller chunks using a while loop and assigning data to variables during the condition check. This can be especially useful for memory management when dealing with very large files.
3. Interactive Menus: When creating interactive menus, this technique can help you manage user interactions and control program flow.
4. Game Development: Assigning values within loop conditions can be valuable in game development, allowing you to update game state variables during each iteration of the game loop.
Considerations and Best Practices
While this approach offers advantages, it's important to be mindful of its potential drawbacks:
Overcomplication: If your loop conditions become overly complex with multiple assignments and logical operators, it can decrease code readability. In such situations, it might be preferable to use separate assignment statements.
Readability: Always prioritize readability. If your loop conditions are too complex, consider using separate assignment statements to enhance clarity.
Code Organization: Maintain code structure and organization. While this technique can reduce code lines, it's important to balance this with clear and maintainable code.
1. Can I assign multiple values within the loop condition?
Yes, you can use multiple assignments within the loop condition by combining them using the and or or operators. For example:
2. Is there a performance difference between assigning values in the condition vs. in the loop body?
In most cases, there is no significant performance difference. However, in specific scenarios involving very complex computations or data manipulation, assigning values within the condition might lead to minor performance gains due to fewer lines of code executed.
3. Are there any limitations to using this technique?
While generally applicable, avoid assigning values within the loop condition for complex calculations or operations that might significantly impact performance.
4. How do I handle multiple conditions with different assignments?
You can chain multiple conditions together using the and and or operators, assigning values to different variables as needed. For example:
5. Can I use this technique with nested loops?
Absolutely. You can use this technique with nested loops to efficiently manage variables within the nested structures. However, pay close attention to the order of assignments to ensure correct logic.
Assigning values to variables within while loop conditions in Python offers a concise and elegant way to manage program flow. By utilizing this technique, you can streamline your code, improve readability, and potentially enhance efficiency. While it provides a valuable tool, remember to exercise caution with complex conditions and prioritize code clarity.
By understanding this approach and its nuances, you can effectively leverage Python's while loops to create more efficient and maintainable code, whether you're validating user input, processing data, or building interactive applications.
Related Posts
- Definition of duplicate keys 14-11-2024 6380
- The Best Nvidia GeForce RTX 40-Series Graphics Cards 14-11-2024 6321
- 10 Common Problems With Microsoft OneDrive and How to Fix Them 14-11-2024 6301
- What to Do If Your Mouse Stops Working 14-11-2024 6293
- Meet Thermonator, a Flame-Throwing Robodog That Can Light Fires, Melt Snow 14-11-2024 6288
Latest Posts
- String vs. Char Array in Java: Understanding the Difference 15-11-2024 824
- Material-UI X Grid: Hidden Columns and Re-rendering Issues 15-11-2024 752
- Appending Dictionaries in Python: Combining Data Structures 15-11-2024 738
- Understanding Beta Coefficients in ANOVA with R and XLSTAT 15-11-2024 527
- Finding a Table by Name in SQL: A Practical Guide 15-11-2024 696
Popular Posts
